zTrace 3.00 (for 64-bit only)
This
Visual Studio 2017 community project, has been totaly reworked to reduce the size down from
91 to
14 Kb.
(with the same technic used to produce the tiny
MBox64 OpenGL visual plugins.)
zTrace is a small DLL utility to display debugging information into a popup window tool, and/or a text file.
It uses a distinct thread to work in parallel of the current application you want to debug.
zTrace is very useful at development time to check whether a program operates properly.
It has been modeled onto the WinDev's Trace API, the original Win32 version was written in PowerBASIC.
I first wrote zTrace to debug my addon graphic DLL tools, but it works also very well with any 32-bit or 64-bit EXE.
When i took the decision to convert WinLIFT and GDImage to C++, it was the first on my list, because I couldn't develop anymore without it.
zTrace uses exclusively the core flat API, that is the only common denominator to all the languages I use, and the only way to get rid of extra dependencies.
The source code is provided in pure SDK coding style like documented into Charles Petzold 5th edition (the SDK coder Bible).
zTrace 64-bit is UNICODE based (32-bit is ANSI).
Syntax to use:zTrace(L"Wide String Information")
Parameter detail:one single unicode (WCHAR) string, holding the information to display in the trace window.
Trace window:• The information passed as parameter is displayed on the next line of the zTrace window.
• The trace window is automatically opened when zTrace is called, by default, this window is opened at the top left corner of the screen.
• The zTrace window shuts down automatically when you close the application being debugged.
zDebug.txt report:• Works exactly like the zTrace window, except that the information is written to a text file.
• The report is automatically created when zDebug is called, it is saved into the same folder than the debugged application.
• zDebug can be used alone or combined with zTrace (when the option is checked in the popup menu).
• zDebug is very handy when the debugged application shuts down unexpectedly or when the application as a short life duration, that won't give you enough time to read what is written in the zTrace window.
• A new fresh zDebug.txt is created each time you start a new zDebug session.
Contextual popup menu (right mouse click on the trace window):
• "Use horizontal scrollbar", show or hide the horizontal scrollbar.
• "Send selection to printer", print the selected lines (or the whole list when none).
• "Copy selection to clipboard", copy the selected lines (or the whole list when none) to clipboard.
• "Clear content", clear the content of the Trace window.
• "Trace window TopMost", open the Trace window on top of all the other windows (including the windows from the other applications).
• "Create zDebug.txt report", the zDebug.txt report is created into the debugged application's folder.
• "Save window coordinates", store the size and position of the zTrace window for the next session.
Screen shot:
Helper function, for EXPLICIT linking:#define long_proc typedef long (__stdcall *zProc)
long zTrace(IN WCHAR* sPtr) {
long nRet = 0;
static HMODULE hDll;
if (hDll == 0) {
if (sizeof(LONG_PTR) == 8) {
hDll = LoadLibrary(L"zTrace64");;
}
else {
hDll = LoadLibrary(L"zTrace32");
}
}
if (hDll) {
long_proc(WCHAR*);
static zProc hProc;
if (hProc == 0) { hProc = (zProc)GetProcAddress(hDll, "zTrace"); }
if (hProc) { nRet = hProc(sPtr); }
}
return nRet;
}
I want to say thank you to
Fred and
James for letting me know how to reduce drastically the size of a 64-bit application.
Settings used to create this tiny 14 Kb 64-bit DLLzTrace Property PagesThe calling Convention for 64-bit is always using
__fastcall (/Gr).
And in 64-bit
no need to use a .def file to avoid the decorated names.
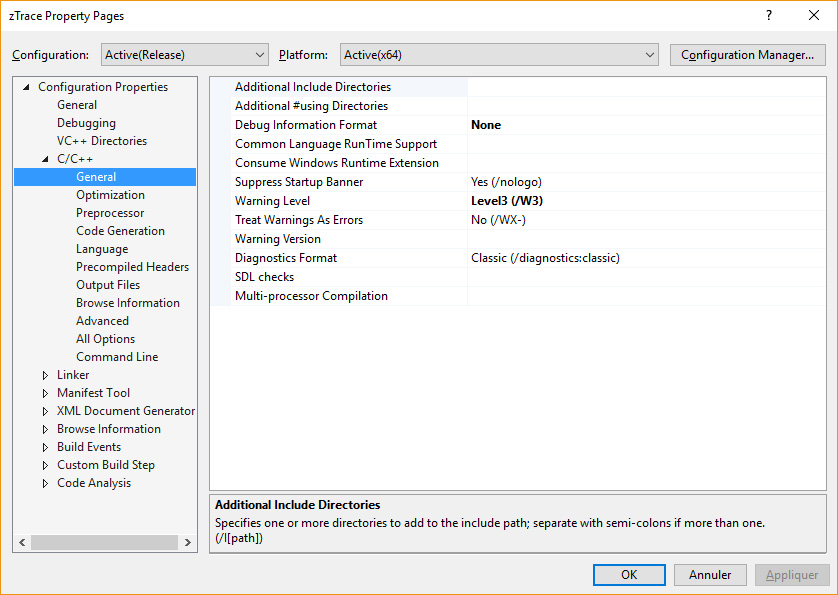
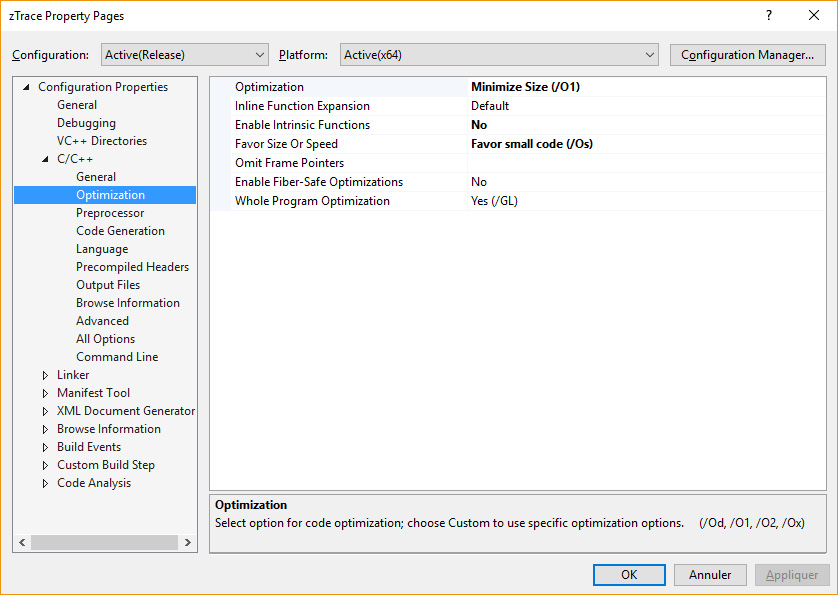
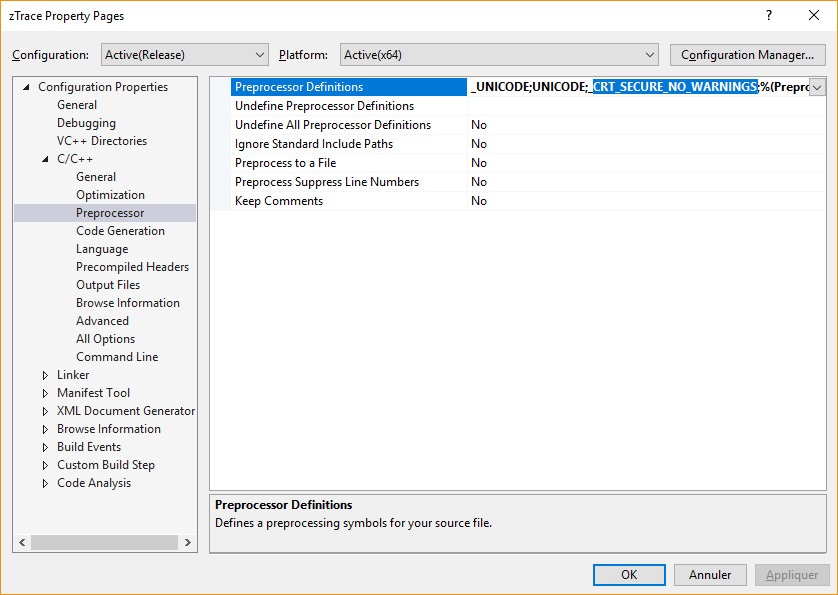
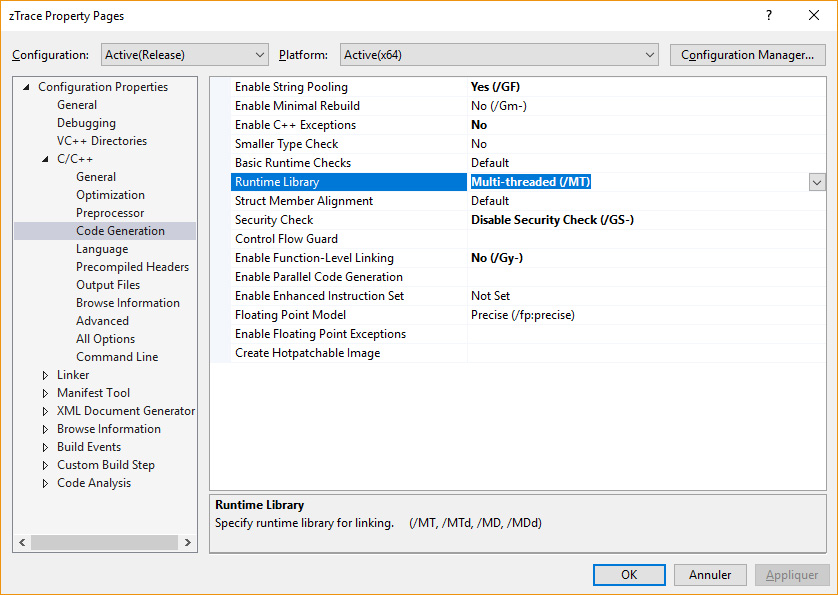
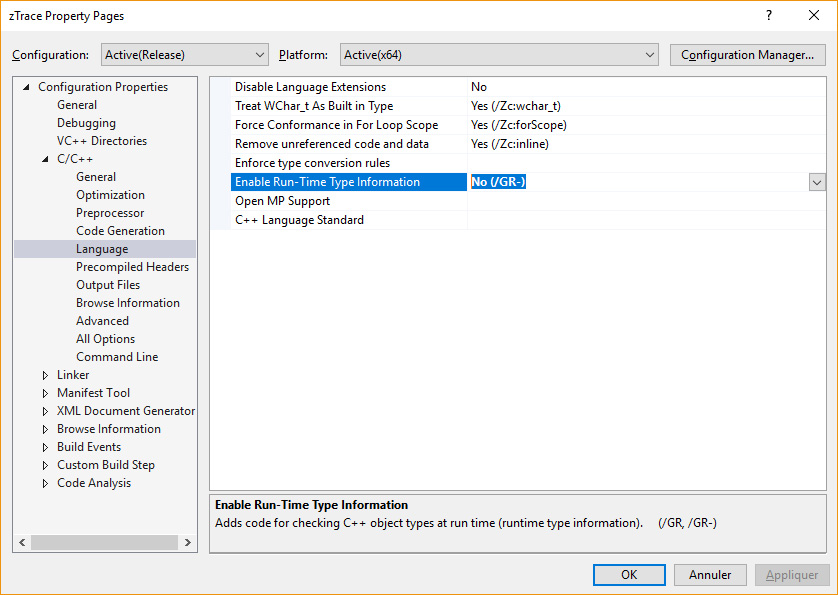
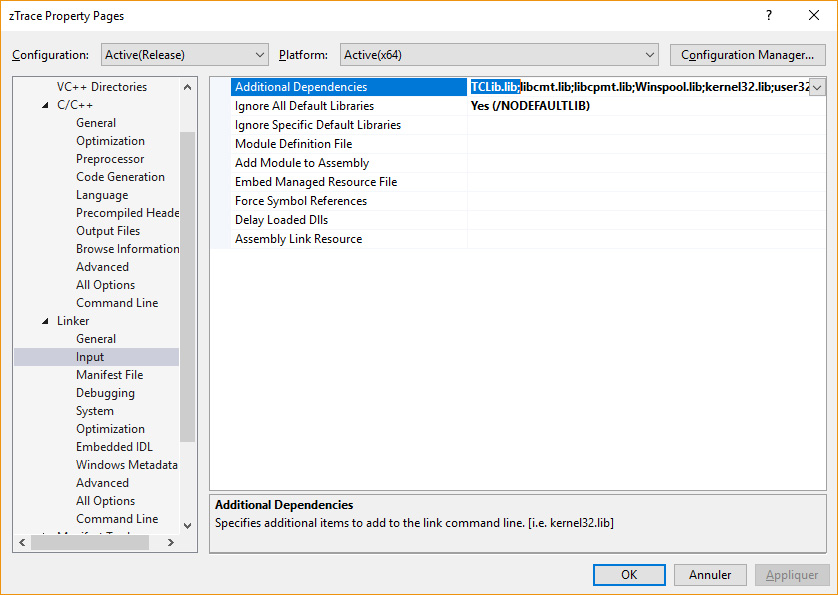
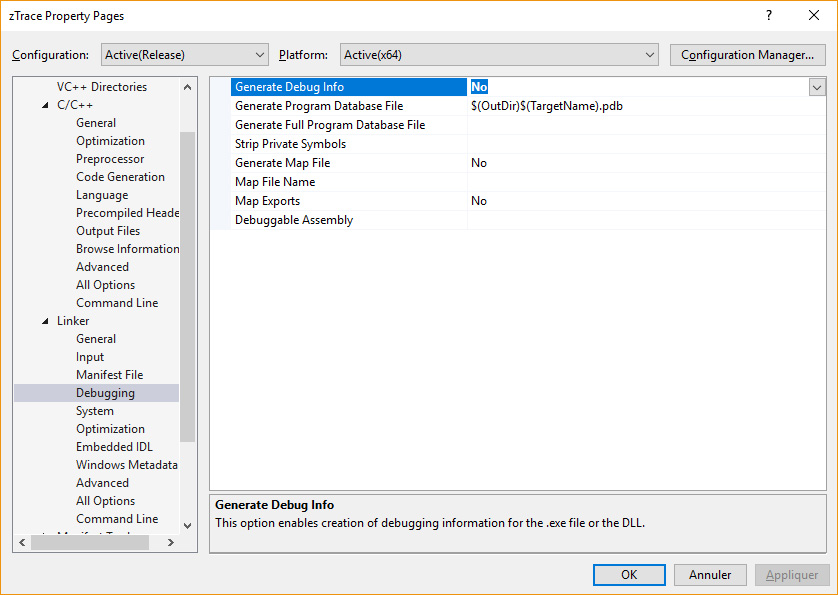
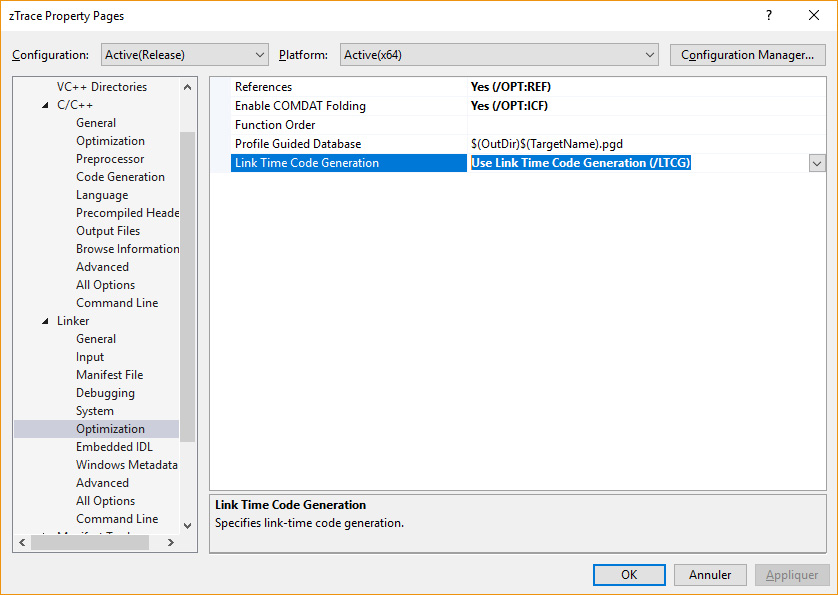
Include + Pragma#include <windows.h>
#include "..\TCLib\Strings.cpp"
#pragma warning(disable: 4996) // remove Unsafe notifications
#pragma warning(disable: 4312) // remove warning C4312: 'type cast': conversion from 'long' to 'HMENU' of greater size
HistorySee it here:
http://www.jose.it-berater.org/smfforum/index.php?topic=3092.004-26-2017Fix:delete [] SelItem;
was unexpectedly missing from the case IDM_Print
Enhancement:New menu option: "
Use Unicode in zDebug.txt"
to switch between ANSI or UNICODE
in case of ANSI, a UTF-8 BOM header is now being used.
UTF-8 BOM is a sequence of bytes (EF BB BF) that allows the reader to identify a file as being encoded in UTF-8.
04-27-2017I have merged Andrei changes into this new version, with:
further code clean up,
new font set for both the listbox window and the printer,
switched back to XP compatibility,
no more TCLib linking that has been removed.
With more features, and further code optimization, the resulting size of the 64-bit Unicode DLL, is now only 11 Kb.